IoT Unlocked: Your Journey to Innovation Starts Now
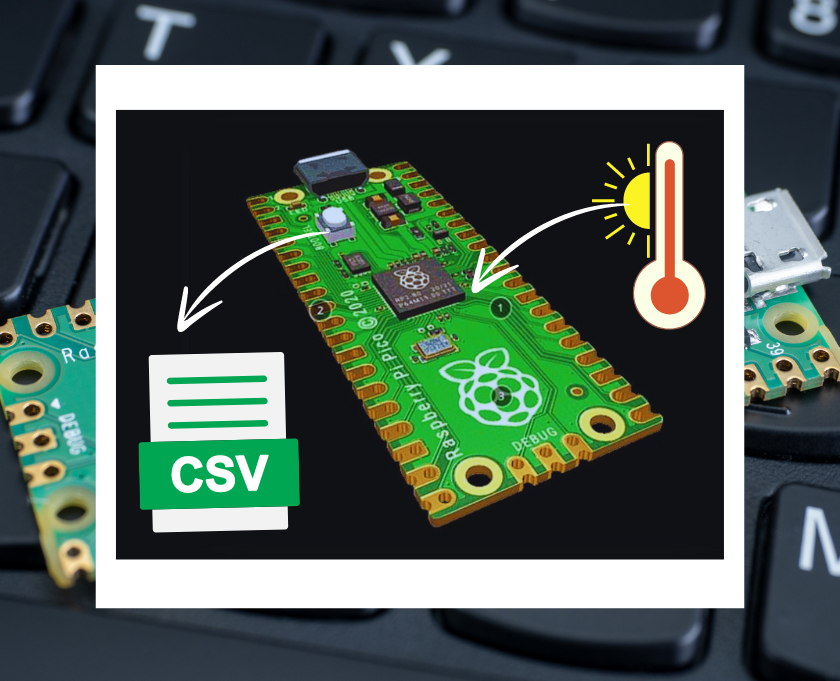
In this step-by-step guide on CSV Data Logger on Raspberry Pi Pico, on this project we captures the temperature readings from an onboard sensor, logs them to a CSV file along with timestamps, and reads back the logged data for analysis or monitoring.
This Sensor Data Logger project is perfect for beginners and enthusiasts alike, this guide will empower you to unlock the full potential of Raspberry Pi Pico in data logging and beyond. Join us to enhance your projects with precise, easy-to-manage sensor data logging capabilities!
Table of Contents
Introduction to Raspberry Pi Pico and CSV Data Logger
The Raspberry Pi Pico is a tiny, fast, and versatile board built using the RP2040 microcontroller chip. With its low cost and high performance, it’s an ideal choice for various projects, including data logging. CSV (Comma-Separated Values) files are a straightforward file format used to store tabular data, making them perfect for logging sensor data over time.
Prerequisites: Read and Write CSV Files with Raspberry Pi Pico
- Raspberry Pi Pico or Pico W
- Micro-USB cable for programming and power supply
- Thonny Python IDE or any Python-enabled environment
- Basic knowledge of Python programming
- External temperature sensor (optional), for this project we are using inbuilt temperature sensor
Setting Up Your Raspberry Pi Pico
To start, connect your Raspberry Pi Pico to your computer using the Micro-USB cable. If you haven’t already, you’ll need to install MicroPython onto your Pico. This can be done through the Thonny IDE, which offers an easy interface to install MicroPython directly.
You can go through: How To Get Started with Raspberry Pi Pico in 2024
Setting Up the Temperature Sensor
First, we initialize the temperature sensor. The Raspberry Pi Pico doesn’t come with a dedicated temperature sensor but has an ADC (Analog to Digital Converter) that can be used to approximate the CPU temperature. This method is more about demonstrating the capability rather than obtaining precise environmental temperatures.
import machine
import time
import os
def read_temperature():
adc = machine.ADC(4) # Use ADC pin GP4
conversion_factor = 3.3 / (65535) # ADC conversion factor
sensor_value = adc.read_u16() * conversion_factor
temperature = 27 - (sensor_value - 0.706) / 0.001721 # Temperature conversion formula
return temperature
Next, we focus on the log_temperature
function, which is responsible for creating a CSV file if it doesn’t exist and appending new temperature readings alongside their timestamps.
Writing Temperature Sensor Data on CSV File
It involves checking if the file exists, creating it with a header if necessary, and then appending each new temperature reading with a timestamp. The code leverages the time.localtime()
function to generate timestamps and formats them for readability.
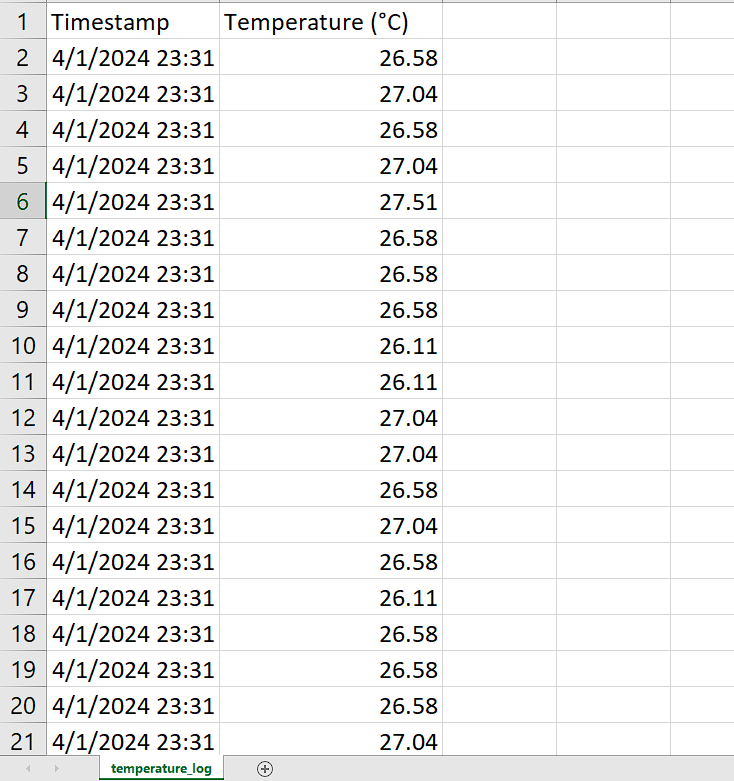
This method allows for a structured and accessible way to log temperature data over time, providing a foundation for data analysis or monitoring applications.
def log_temperature():
file_name = "temperature_log.csv"
timestamp = time.localtime()
temperature = read_temperature()
timestamp_str = format_timestamp(timestamp)
# Check if CSV file exists, create it with a header if it doesn't
if not file_name in os.listdir():
with open(file_name, 'w') as file:
file.write('Timestamp,Temperature (°C)\n')
# Append temperature data to the CSV file
with open(file_name, 'a') as file:
file.write('{},{:.2f}\n'.format(timestamp_str, temperature))
The format_timestamp
function neatly formats the timestamp into a human-readable string for easier analysis.
def format_timestamp(timestamp):
year, month, day, hour, minute, second, *_ = timestamp
timestamp_str = "{:04d}-{:02d}-{:02d} {:02d}:{:02d}:{:02d}".format(year, month, day, hour, minute, second)
return timestamp_str
Reading Logged Temperature Data from CSV
For analyzing or simply checking the logged data, the read_temperature_log
function reads the CSV file, skipping the header, and parses each line to collect timestamps and temperature readings.
def read_temperature_log():
file_name = "temperature_log.csv"
temperature_data = []
if file_name in os.listdir():
with open(file_name, 'r') as file:
next(file) # Skip header
for line in file:
parts = line.strip().split(',')
if len(parts) >= 2:
timestamp = parts[0].strip('(') # Clean up timestamp
temperature = float(parts[1])
temperature_data.append((timestamp, temperature))
else:
print("Invalid line:", line)
return temperature_data
Running the CSV Data Logger
Finally, the script demonstrates a simple loop where temperature readings are logged every 2 seconds, up to 5 readings, after which the logged data is printed out before resetting the counter and repeating the process.
counter = 0
while True:
counter += 1
if counter <= 5:
log_temperature()
else:
print(read_temperature_log())
counter = 0
time.sleep(2)
CSV Data Logger: Complete Code
You can check out this GitHub Gist or copy from below code snippet:
import machine
import time
import os
# Function to read temperature sensor
def read_temperature():
adc = machine.ADC(4) # Use ADC pin GP4
conversion_factor = 3.3 / (65535) # ADC conversion factor
sensor_value = adc.read_u16() * conversion_factor
temperature = 27 - (sensor_value - 0.706) / 0.001721 # Convert sensor value to temperature (formula may vary)
return temperature
# Function to log temperature data to CSV file
def log_temperature():
file_name = "temperature_log.csv"
timestamp = time.localtime()
temperature = read_temperature()
print(temperature)
timestamp_str = format_timestamp(timestamp)
# Check if CSV file exists, if not create it with header
if not file_name in os.listdir():
with open(file_name, 'w') as file:
file.write('Timestamp,Temperature (°C)\n')
# Append temperature data to CSV file
with open(file_name, 'a') as file:
file.write('{},{:.2f}\n'.format(timestamp_str, temperature))
def format_timestamp(timestamp):
year, month, day, hour, minute, second, *_ = timestamp
timestamp_str = "{:04d}-{:02d}-{:02d} {:02d}:{:02d}:{:02d}".format(year, month, day, hour, minute, second)
return timestamp_str
# Function to read temperature data from CSV file
def read_temperature_log():
file_name = "temperature_log.csv"
temperature_data = []
# Check if CSV file exists
if file_name in os.listdir():
with open(file_name, 'r') as file:
# Skip header line
next(file)
# Read each line and parse data
for line in file:
parts = line.strip().split(',')
if len(parts) >= 2:
timestamp = parts[0].strip('(') # Remove any extra characters from the timestamp
temperature = float(parts[1])
temperature_data.append((timestamp, temperature))
else:
print("Invalid line:", line)
return temperature_data
counter = 0
# Example usage: Log temperature every 5 seconds
while True:
counter += 1
if counter <=5:
log_temperature()
else:
print(read_temperature_log())
counter = 0
time.sleep(2)
Conclusion
This temperature logging project is a great starting point for anyone looking to get hands-on with the Raspberry Pi Pico and delve into data logging and sensor reading. With this foundation, you can expand the project by integrating different sensors, enhancing the data logging capabilities, or even setting up a web server to monitor the readings in real time. Happy coding!
FAQs:
How do I ensure the accuracy of temperature readings from the onboard sensor?
While the onboard sensor of Raspberry Pi Pico provides approximate temperature readings, for more precise measurements, consider calibrating the sensor or using external sensors with higher accuracy.
What if I want to visualize the logged data in graphs or charts?
You can export the CSV data to popular data analysis tools like Python’s Pandas or Matplotlib libraries to visualize the temperature trends over time in interactive graphs or charts.
Can I set up automated alerts based on temperature thresholds?
Yes, by incorporating conditional statements into the code, you can trigger alerts or notifications when temperature readings exceed predefined thresholds. This functionality can be useful for early warning systems or remote monitoring applications.
How does the temperature sensor work on Raspberry Pi Pico?
Raspberry Pi Pico doesn’t have a dedicated temperature sensor. Instead, it uses the onboard ADC (Analog to Digital Converter) to approximate the CPU temperature. This method converts the analog temperature signal into a digital value that can be read by the microcontroller.
Can I modify the code to log data at different intervals?
Yes, you can adjust the time.sleep() function in the code to control the logging interval. For example, setting time.sleep(60) would log temperature data every 60 seconds, while time.sleep(3600) would log data every hour.
Read also: How to Build Your Own Web Server on Raspberry Pi Pico W and Phew: A Step-by-Step Guide